Hello everyone.
First of all, sorry for my english. This is my first post here, so please be patient with me.
I had an idea recently, and searched the internet for a forum like this one to discuss it.
It is quite simple:
Take a NES rom file, and reproduce it taking every 4 bits (a pixel in NES) making that same nibble (a nibble is a set of 4 bits) appear 16 times intead of just one time. Each pixel now would be a matrix of 4 x 4 pixels.
After this, take an open source emulator and change the code in a way to make it have a screen of 1024 X 960 (four times the original 256 x 240).
Additionally, in every graphic instruction, make the emulator take the tiles from the new rom file (the one with 16x nibbles).
Of course, initially the graphics would look the same. But after some tile editing, we could have graphics like this one, for example:
Instead of looking like this:
Obviouly, there are people out there that could make it look far better, but it is just an example. Using black pixels (or darker colors) to make shades would be beautiful.
The idea is to have fan made HD remakes of old classics, with the help of everyone.
If there are NES limitations that would make it impossible to achieve, please tell me.
I am not a programmer (at least, not an emulator programmer), so if anyone wish to help, it would be awesome.[/img]
This comes up again every once in a while.
Enhancing graphics in a NES emulator wouldn't be all that hard to do, but I don't know of any emulators that actually support it yet.
But one way to accomplish this is to use replacement graphics. Look up the original tile's graphics, and overlay a replacement tile instead. The replacement graphic would not necessarily be constrained to the size of the original tile, it could be a few pixels larger in all directions. And it would not be restricted to the original palette, it could use a larger palette chosen based on which original NES palette it is using. I'd recommend 16 color or 256 color replacement graphics, so you can still do palette-swaps.
Games that do pixel effects on the game's graphics would be a problem, since the tiles wouldn't match anymore, but those are rare.
For background tiles, replacement graphics that are bigger than the original tile would be more of a problem, since you would need to define an order for tiles to be drawn. Maybe make some tiles higher priority than others, and if there's a tie, tiles to the right and tiles below win.
Extra frames of animation? Not likely. For that you need to modify the programming of the original game.
Some masking effects would still need to be done. Super Mario 3 puts low-priority sprites behind tiles so that later sprites get masked off. This would still need to be simulated even with replacement graphics.
Then there's the sprite limit. Normally a nuisance that just gets in the way, makes ugly blinky sprites everywhere. But some games do use the sprite limit for masking effects to hide other sprites in the area, such as Zelda 1 or Gremlins 2. Those can be detected.
Games that use CHR ROM could do this, but games that use CHR RAM would need to identify each tile through some sort of hash table on 16-byte regions of the pattern table. It would not work for games that dynamically update background tiles, such as Videomation (drawing program), Qix (drawing program with enemies), Hatris (objects in background not aligned to 8x8 grid), 3D Block (likewise unaligned), Shanghai 2 (same), and text boxes in Super Bat Puncher and the Action 53 menu.
But if anyone wants to work on implementing hi-res texture packs, let me know, and I'll whip up a hi-res copy of Thwaite 0.03's CHR ROM for testing.
Do you guys know an open source emulator that would be the easiest to use for this?
I have some knowledge in Visual Basic, Basic, Pascal, C and Java.
If we could get it to work, it would be good to have a modified version of Tilificator to edit the graphics.
joabfarias wrote:
Do you guys know an open source emulator that would be the easiest to use for this?
I have some knowledge in Visual Basic, Basic, Pascal, C and Java.
If we could get it to work, it would be good to have a modified version of Tilificator to edit the graphics.
Sounds more like you're wanting an emulator with a built in
hq2x magnification filter.
cpow wrote:
Sounds more like you're wanting an emulator with a built in
hq2x magnification filter.
No, it is not.
The human brain/eye can reconstruct a picture far better than any filter, 2xSAI or HQ3x are just workarounds.
If you can make a filtered image look as good as what I did above, I will shut my mouth.
joabfarias wrote:
cpow wrote:
Sounds more like you're wanting an emulator with a built in
hq2x magnification filter.
No, it is not.
The human brain/eye can reconstruct a picture far better than any filter, 2xSAI or HQ3x are just workarounds.
If you can make a filtered image look as good as what I did above, I will shut my mouth.
That was kind of my point. I couldn't distinguish the result you're looking for from the h2qx results I linked to. Maybe I'm blind. I'll shut my mouth.

cpow wrote:
I'll shut my mouth.

Sorry if I was uneducated, cpow. It was not my intention.
I believe that better pixel artistis could make the example I made look superb, using shading and other tecniques, even with the NES color limitations.
I will see if I can adjust the code of HalfNes (a java opensource NES emulator) to make what I want.
If any of you believe there are easier ways of doing it, please tell me.
Sounds pretty doable for CHR-ROM games. CHR-RAM probably not. Extra colours would be hard to reconcile with palettes, probably best to stick with the original 4-colour versions.
You could probably include the HD CHR file alongside the ROM, like subtitle files for movies. The regular CHR inside the ROM would be used internally, and at render time replace the operation that renders a pixel with an operation that renders 4 pixels. Should hopefully be pretty straightforward.
Why has nobody done this? I suspect most people who want to emulate Megaman are pretty cool with how many pixels he has. Probably some people would be interested in this, but the majority I think just wants it to look like an NES.
rainwarrior, you are right when you say that some people want it to look like the original NES.
But when the community begins to remake the graphics with REALLY GOOD work (not like the example I made), I am sure that it will be something worth of attention.
Besides, I think that the graphics replacement will take much less CPU power than magnification filters. If this is true, we can start to make the original pixels 6x6 or 8x8 large, and it will bring much more artistic possibilities.
rainwarrior wrote:
Sounds pretty doable for CHR-ROM games. CHR-RAM probably not.
Unless you map each distinct 8x8 pixel tile in CHR RAM to a tile in the replacement texture pack. That's what I meant by a hash table. For anything that doesn't generate CHR data at runtime, the hash table method should be nearly as reliable as CHR ROM.
Quote:
Extra colours would be hard to reconcile with palettes, probably best to stick with the original 4-colour versions.
I can think of two ways to work around this. One involves storing a 15-color palette for each possible 3-color palette. Another involves generating a palette like so:
0-3: Old colors
4-7: Black and colors 1-3 mixed 50:50 with black
8-11: White and colors 1-3 mixed 50:50 with white
13-15: Colors 1 and 2, 1 and 3, and 2 and 3 mixed 50:50 (for internal antialiasing)
Should I draw a picture of what such a palette might look like?
So we'll need a tool which matches original tiles to a sprite sheet image, then a tool to help the user select which image in a sprite sheet corresponds to another image in an enhanced sprite sheet.
Because palettes will not exactly match between CHR data and sprite sheets, do matching by unique colors. You may need to edit a sprite sheet if the author added unnecessary stuff such as outlines.
Or just work with tile dumps. You have the original tile dump, then another enlarged or enhanced version of the tile dump. Correspondence is done entirely by position.
For games that use CHR RAM, savestates can help you get tile data.
This is starting to get big.
A moment will come when somebody will want to replace Princess Daisy with a bikini babe.
I think this is a really cool idea.
It reminds me of Spec256 - an emulator that together with hacked games could render old ZX Spectrum games in 256 colors. A video of it in action can be seen here:
http://www.youtube.com/watch?v=xGN59bA1n3M
jayminer wrote:
Spec256
I wonder how they managed to tell the game objects apart, since AFAIK the Spectrum only has a single graphics plane (i.e. no hardware sprites).
On the NES, it would be better if the same tiles could be mapped to different upscaled bitmaps depending on their palette, otherwise it would be hard to make good looking high-resolution bushes and clouds in SMB, for example. Enemies that are just palette swaps could benefit from this too, and receive extra details.
tokumaru wrote:
On the NES, it would be better if the same tiles could be mapped to different upscaled bitmaps depending on their palette, otherwise it would be hard to make good looking high-resolution bushes and clouds in SMB, for example. Enemies that are just palette swaps could benefit from this too, and receive extra details.
I think that the palette swaps are the main reason to keep the same colors used originally. It would save a lot of work.
With more pixels, it is easier to make shades using the same colors.
Perhaps it will be possible to use some dithering filter in the future, and make images like this one:
Using 3 colors to do this would make awesome images.
Dithering and 3 colors? I thought this was about expanding NES graphics to new high-color graphics.
joabfarias wrote:
But after some tile editing, we could have graphics like this one, for example:
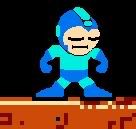
I'll take the original any day of the week. Just an opinion (doesn't represent the world or anything): I wish people (including companies) would leave classic games alone. Trying to make money off nostalgia irritates me, but that's probably because "remade" classic games tend to be crap (yeah, including that Bionic Command remake), art and all. It's a sign of the times: people just want to make a quick buck rather than actually come up with something new/inventive.
Dwedit wrote:
Dithering and 3 colors? I thought this was about expanding NES graphics to new high-color graphics.
Well, putting more colors would be beautiful, but I think it would be far more difficult. How would we manage the palette swaps?
If we keep the same colors, everything will work.
Imagine a MegaMan body sprite with several colors. After a weapon change, the palette will swap. The original colors will swap normally, but not the additional colors. How you intend to make it work?
You look up a different 256 or 16 color palette for each possible 3-color palette. Tepples suggested a way to auto-generate a 16-color palette: darker and brighter versions of the 3 colors, and blended colors. But you don't have to use it, you can just use a custom-made palette.
koitsu wrote:
joabfarias wrote:
But after some tile editing, we could have graphics like this one, for example:
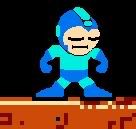
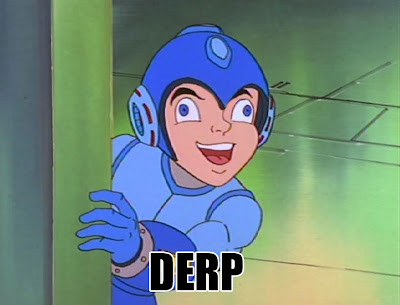
I'll take the original any day of the week. Just an opinion (doesn't represent the world or anything): I wish people (including companies) would leave classic games alone. Trying to make money off nostalgia irritates me, but that's probably because "remade" classic games tend to be crap (yeah, including that Bionic Command remake), art and all. It's a sign of the times: people just want to make a quick buck rather than actually come up with something new/inventive.
koitsu, I made that image in just a few minutes, and I don't have any experience in pixel art. I am very certain that people who know how to do this (and love the game) would make it look beautiful.
I did not understand why you are talking about money. Everything would be made purely by passion, by gamers and for gamers.
Dwedit wrote:
You look up a different 256 or 16 color palette for each possible 3-color palette. Tepples suggested a way to auto-generate a 16-color palette: darker and brighter versions of the 3 colors, and blended colors. But you don't have to use it, you can just use a custom-made palette.
Sorry, now I understand. You are right, it would really look beautiful.
But we have to be sure that everything will run in an average CPU.
The quantity of pixels has reached almost a million. Of course, we can use 2x2 matrixes with more colors instead of 4x4, with a final resolution of 512 x 480.
Yeah, 2x pixel graphics are better than anything else IMO. Look at Sonic. Sonic 4 on 360 doesn't even phase me, while the original Sonic is awesome to me.
3gengames wrote:
Sonic 4
Poor example, that game just plain sucks.
tokumaru wrote:
3gengames wrote:
Sonic 4
Poor example, that game just plain sucks.
graphics don't, game does.
Couldn't this be done with Lua scripting? It would seem to be the most flexible way, but I'm not sure if any emulators with Lua support creating higher res graphics than the nes resolution.
http://www.youtube.com/watch?v=IvDXZmS2MAU
Similar things had been done in a really old arcade game emulator (for DOS) called EmuDX, in which you can replace the graphics and sound clips with new ones. There was also a
certain SMS emulator that popped up a few years back in which you can replace its graphics by high resolution ones (read the tutorials; it's similar to what you want to do here).
So all this is possible (provided some people make the tools) but honestly unless the enhanced graphics are really good enough I'll stick to the original. Personally I think a much more worthwhile enhancement to Famicom graphics is to increase the colours (like from 4 colour to 16 colour for each tile; but then games with palette effects would need more attentions) than the resolution.
Hmm, perhaps instead of 4-colours it could be a 4-bit palette index multiplied by a 4-bit greyscale value, or perhaps an HSV colour offset from the palette colour. That would allow some extra colours without making palette-swaps a hassle. (Probably would need a dedicated tool for coming up with HSV offsets; I don't know if this exists anywhere.)
I like Tepples' idea of hashing, or having some sort of lookup based on the tile's position on the CHR page and its content, to be able to deal with CHR-RAM easily.
Just creating a CHR-ROM files that is 4x the size of the regular CHR-ROM though would be about the simplest possible file format, and have relatively low ambiguity though.
On another note, has anyone ever made an automated sprite ripper for NES? I was thinking you could play the game normally, and any block of sprites that are placed contiguously onscreen could be automatically rendered to an output bitmap (filtering any already used). It'd come up with a ton of false positives (e.g. two characters stuck together) but it would probably do a pretty good job otherwise. Maybe sprite ripping isn't all that hard to accomplish to begin with though.
The automatic sprite ripper would really come in handy, because it also contains the tile numbers that make up that sprite.
I tried making a tool that matched sprite pieces on a sprite sheet back to CHR data, and it's not quite ready yet. For example, it doesn't like overlaid sprites that cover up things. Megaman's helmet covers up one pixel of his face, and it's not matching it. Also, Megaman 2's graphics have a couple of duplicate tiles.
But anyway yeah, automatic sprite ripper, then manually correspond the images to enhanced images. Easy to take it from there, and get the replacement graphics ready.
I remember that the DOS version of NESticle allowed the user to edit the sprites during emulation. It was not a very good interface, but was pretty easy to change things.
If the tool to redesign the sprites could be inside the emulator like in NESticle, with the results being show in real time, it would be really good.
tepples wrote:
Quote:
Extra colours would be hard to reconcile with palettes, probably best to stick with the original 4-colour versions.
I can think of two ways to work around this. One involves storing a 15-color palette for each possible 3-color palette. Another involves generating a palette like so:...
A couple of months ago, I played around with a similar concept. Here are a couple examples:
For example, for BGs you have an additional 12 colours available to be used. You could fill them with a preset palette that you choose so all tiles can use the extra colours at any time. However, for re-used tiles (think SMB1 clouds are also bushes), re-touching tiles with preset colours doesn't work when they're used multiple times with different palettes.
Another option is to interpolate the extra 12 colours from each 4-entry NES palette.
ex:
A routine to mix the extra colours from the base 4 shouldn't be too tough...
Also, when the game changes palettes, the relationships between all the colours stays logical:
Just an idea... never mind the gaudy over-colouring of my sprite example.
joabfarias wrote:
koitsu, I made that image in just a few minutes, and I don't have any experience in pixel art. I am very certain that people who know how to do this (and love the game) would make it look beautiful.
Sorry, I guess my intentions were misunderstood, and that's understandable. So let me explain them:
My point wasn't that your art sucked. My point was that you want to, in a bizarre way, "make existing classic games look different than how they were intended". You aren't talking about making a new game, or some spin-off (and like I said most of those I've seen in the past 20 years have been horrible), you're talking about trying to improve upon something that doesn't need improvement; and I'm intentionally ignoring the technical limitations as well.
joabfarias wrote:
I did not understand why you are talking about money. Everything would be made purely by passion, by gamers and for gamers.
Which doesn't give it any more or any less merit.
My point, plain and simple:
leave classic video games alone. They do not need to be improved, changed, enhanced, or modified. I don't want my NES games in HD. I want my NES games looking the way they were intended during their creation.
I'm glad people are talking about graphics and how to make things that look neat or better, yadda yadda, it's all interesting but again,
leave the existing games alone. Old crotchety men like me have grown very tired of people trying to "enhance" nostalgia; it's no longer nostalgia when you fuck with it. All that happens is said nostalgia gets ruined.
koitsu, but if you don't want to play HD remake, you can just ignore it, correct? There are people with different opinions, who may enjoy it. It may even be not too bad, actually - check
Monkey Island 2 HD remake (it allows to switch between the original and remake with a key at any point, by the way).
The idea to make an emulator that replaces graphics with hi-res and sound with MP3's is old and was proposed many times. I recall it from ~2004, I think. But still no one is really got up to the task, and I think that lack of artists would be a real showstopper. Also, to 'sell' the idea to people you certainly shouldn't use examples like in the first post, it only spoils the idea as it looks inferior to the original.
Well, koitsu, you made your point. Now, if you excuse us, we will continue our talk. Nothing personal, just a plain case of freedom of thought.
I am trying to make some changes in the source code of Nintendulator. If anyone knows a good C compiler that could correctly recompile the thing, suggestions will be welcome.
Shiru wrote:
The idea to make an emulator that replaces graphics with hi-res and sound with MP3's is old and was proposed many times. I recall it from ~2004, I think. But still no one is really got up to the task, and I think that lack of artists would be a real showstopper. Also, to 'sell' the idea to people you certainly shouldn't use examples like in the first post, it only spoils the idea as it looks inferior to the original.
Yeah it's another catch-22. Programmers don't want to implement it in emulators until at least one hi-res pack is available, artists don't want to make the hi-res pack unless emulators support it. For this to happen eventually somebody is going to have to take the plunge.
As for what compiler to use to compile Nintendulator, Visual Studio is pretty much the only choice.
I was never a fan of this idea.
It wouldn't look as good as people imagine it. It might work for backgrounds, but the sprites would look horribly out of place mainly because animation restrictions are still there.
Megaman, for example. Even with souped up graphics, his walking animation is only 3 frames. With NES graphics that looks natural. With "HD" graphics it would look absurd.
Link in the original Legend of Zelda has a 2 frame walking animation.
Link in Zelda 3 had what... 8 frames? Can you imagine how ridiculous Zelda 3 link would look if you cut that animation down to 2 frames?
Also a lot of games recycle tile graphics for multiple frames. Final Fantasy, for example, draws its character graphics with a 2x3 arrangement of sprites. However, when walking, only the bottom tiles animate.. the top 4 tiles are the same as the "standing" graphics. Megaman would be another example of this... when walking, not all of his tiles change each frame.
It also breaks effects. If you throw out the NES palette and use true color images, what do you do about palette animations? How do you tell the difference between red koopa shells and green ones?
It also changes the emulation aspect of things. For an emulator to be really accurate, it has to fetch and process chr data at very specific times. To handle "HD" data, you'd basically have to create an entirely separate PPU emulator. And couldn't that also change the behavior of potentially game altering effects like sprite-0 hit?
^I think a Lau script overtop of the nes emulation would be able to overcome most, if not all of those limitations, though it would need to be customized to each game. I think any solution would have to be customized to each game anyway though.
Disch wrote:
I was never a fan of this idea.
It wouldn't look as good as people imagine it. It might work for backgrounds, but the sprites would look horribly out of place mainly because animation restrictions are still there.
Megaman, for example. Even with souped up graphics, his walking animation is only 3 frames. With NES graphics that looks natural. With "HD" graphics it would look absurd.
Link in the original Legend of Zelda has a 2 frame walking animation.
Link in Zelda 3 had what... 8 frames? Can you imagine how ridiculous Zelda 3 link would look if you cut that animation down to 2 frames?
Also a lot of games recycle tile graphics for multiple frames. Final Fantasy, for example, draws its character graphics with a 2x3 arrangement of sprites. However, when walking, only the bottom tiles animate.. the top 4 tiles are the same as the "standing" graphics. Megaman would be another example of this... when walking, not all of his tiles change each frame.
It also breaks effects. If you throw out the NES palette and use true color images, what do you do about palette animations? How do you tell the difference between red koopa shells and green ones?
It also changes the emulation aspect of things. For an emulator to be really accurate, it has to fetch and process chr data at very specific times. To handle "HD" data, you'd basically have to create an entirely separate PPU emulator. And couldn't that also change the behavior of potentially game altering effects like sprite-0 hit?
You pretty much have to design your new graphics for many different palettes, since palette swaps are a reality. Think of it as replacing NES graphics with SNES-style graphics, then possibly increasing the resolution as well. SNES games used palette swaps too.
Using 16-bit or 24-bit truecolor graphics isn't a great idea at all. I was never suggesting anything like that. Maybe for title screens or something...
I hadn't even thought of different graphics depending on palettes until somebody brought up the clouds and bushes of SMB1. Maybe for specific palettes, they could use different images.
As for the question about sprites being only partially animated, there are two possible approaches to sprite replacement.
You could do a simple tile replacement, where the new tiles are constrained to the size of the old tiles. Then you would need to match the original art style, and work around how the original sprites were animated.
The other approach is to allow replacement "tiles" to be much bigger that the source tile, and can be drawn at an offset location. So you could blank out the parts that animate the wrong way, and make the other sprites bigger so the entire image is covered.
But if you are trying to make new graphics for a classic game, you probably have a lot of respect for the source material, so you are probably more likely to try to emulate how the original game chose to animate its graphics.
The Mega Man walk cycle is downright iconic. Even when it's actually
Coach Z doing a hip-hop dance.
As for emulation, you don't have to change anything at all. You still do enough PPU simulation for accurate sprite 0 hits and Zapper emulation using the original character data. Then you have another engine draw the replacement graphics instead of the original graphics.
Oversized replacement background tiles might be a little tricky, since they can be bankswitched in and out of existence while the frame is rendering, and the tiles can also be scrolled around with wavy background effects. But I think there's a good enough way to handle them.
joabfarias wrote:
If the tool to redesign the sprites could be inside the emulator like in NESticle, with the results being show in real time, it would be really good.
No it wouldn't. The editing tools wouldn't be good, so the result would end up looking like your example (which everyone already made clear they didn't like). I'm all for the use of MSPaint for pixel art, but high-resolution sprites are a different thing. To make decent graphics you'll need decent software (Photoshop, Illustrator, etc.), there's no reason to waste time trying to implement drawing tools into an emulator.
Disch wrote:
If you throw out the NES palette and use true color images, what do you do about palette animations? How do you tell the difference between red koopa shells and green ones?
Dwedit wrote:
I hadn't even thought of different graphics depending on palettes until somebody brought up the clouds and bushes of SMB1. Maybe for specific palettes, they could use different images.
Yeah,
I already mentioned that in this thread, but apparently people didn't pay much attention.
Disch wrote:
It also changes the emulation aspect of things. For an emulator to be really accurate, it has to fetch and process chr data at very specific times. To handle "HD" data, you'd basically have to create an entirely separate PPU emulator. And couldn't that also change the behavior of potentially game altering effects like sprite-0 hit?
Like Dwedit said, the emulation will remain untouched. As I see it, the HD engine would peek at the emulation state and use palette, pattern, scroll, etc. information to render its image.
The idea is exactly like this, keep all instructions that are not graphics related untouched.
The games would look 512x480 (or more), but would "feel" like the old 256x240.
It would be impossible, for example, to scroll the screen in just one pixel to the left, since there are not really 512 columns but 256 "sets" of 2 columns.
Graphics resolution is not a problem. Many emulators can and usually do run at higher resolution than the NES. This allows some of the filters (ex. hq2x) to work.
Gilbert wrote:
Similar things had been done in a really old arcade game emulator (for DOS) called EmuDX, in which you can replace the graphics and sound clips with new ones. There was also a
certain SMS emulator that popped up a few years back in which you can replace its graphics by high resolution ones (read the tutorials; it's similar to what you want to do here).
So all this is possible (provided some people make the tools) but honestly unless the enhanced graphics are really good enough I'll stick to the original. Personally I think a much more worthwhile enhancement to Famicom graphics is to increase the colours (like from 4 colour to 16 colour for each tile; but then games with palette effects would need more attentions) than the resolution.
Just now I could see your links. HISMS is exactly what I had in mind. My point is: the NES community is far greater than the Sega Master System one, so it is more likely to have good pixel artists.
The graphics that are posted as example of Alex Kidd are really bad, but shows well the emulator capabilities.
Screenshots:
http://forums.ngemu.com/showthread.php?t=119198
http://hisms.orgfree.com/tutorial/index.html
At this point, with the complexity, it would almost be "better" to make game-specific emulators that run the original game logic underneath (for 100% accurate gameplay) but use knowledge of the game's engine specifics to generate a display using higher-quality assets (if not well-done 3D models). I'm not talking making 2D games 3D but rather 2.5D.
LocalH wrote:
At this point, with the complexity, it would almost be "better" to make game-specific emulators that run the original game logic underneath (for 100% accurate gameplay) but use knowledge of the game's engine specifics to generate a display using higher-quality assets (if not well-done 3D models). I'm not talking making 2D games 3D but rather 2.5D.
You mean like the graphics in "Operation Ragnagard" (Neo Geo)? That would be possible.
I will start to mess around with the source code of Nintendulator (finally I got a copy of Visual Studio). I plan to write the utility to convert the rom file turning every pixel in four pixels (I will focus on the colors issue later).
Do any of you guys know if it would do any damage if I "deinterlace" the graphics (usually, the first and the second bit come in different planes -
http://wiki.nesdev.com/w/index.php/PPU_pattern_tables)? I think it would be easier to work this way.
joabfarias, how about this:
--------------------
$0000-$1FFF = (Blank, Unused in enhanced games)
$2000-$2FFF = Nametables
$3000-$3EFF = Unknown
$3F00-$3F1F = Palette #0
$3F20-$3F3F = Palette #1
$3F40-$3F5F = Palette #2
$3F60-$3F7F = Palette #3
$4000-7FFF = Enhanced CHR
Palette Format:
16 for Backgrounds first, then 16 for Sprites = 32 * 4 = 128 colors, You can select out of 64 (or 256) colors depending on what implentation is used
Enhanced CHR only need two transparency areas: $x0, where x is an array of 0-7, the zero at the end of the value is the fixed value used in the transparency value!
There is an emulator able to replace graphics in NES games. It's called "MyNES" (
http://sourceforge.net/projects/mynes/files/ )
I wanted to use this "HiRes" feature desperately but it was too hard to me and I never tried again...

There are pictures of some experiments with a 'Dragon Ball Z' game:



And there's even a video on
http://youtu.be/haGHtKWmj5M
The latest versions of My NES no longer supports it unfortunately, and may never come back!
There should be a better way than doing it in a double-pixeled bitmap mode anyways.
Hamtaro126 wrote:
The latest versions of My NES no longer supports it unfortunately, and may never come back!
There should be a better way than doing it in a double-pixeled bitmap mode anyways.
Oh, sad news

I had never heard about HiSMS until reading this topic.
I'm enjoying a lot to change Sega Master System graphics - so I'd love to see something similar (and as easy to use) done to my beloved NES someday.
By the way this is my modification test with "Alex Kidd in Miracle World":
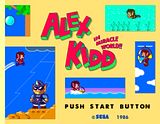
Original screen:
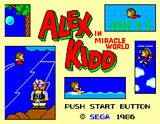
Macbee wrote:
Hamtaro126 wrote:
The latest versions of My NES no longer supports it unfortunately, and may never come back!
There should be a better way than doing it in a double-pixeled bitmap mode anyways.
Oh, sad news

I had never heard about HiSMS until reading this topic.
I'm enjoying a lot to change Sega Master System graphics - so I'd love to see something similar (and as easy to use) done to my beloved NES someday.
By the way this is my modification test with "Alex Kidd in Miracle World":
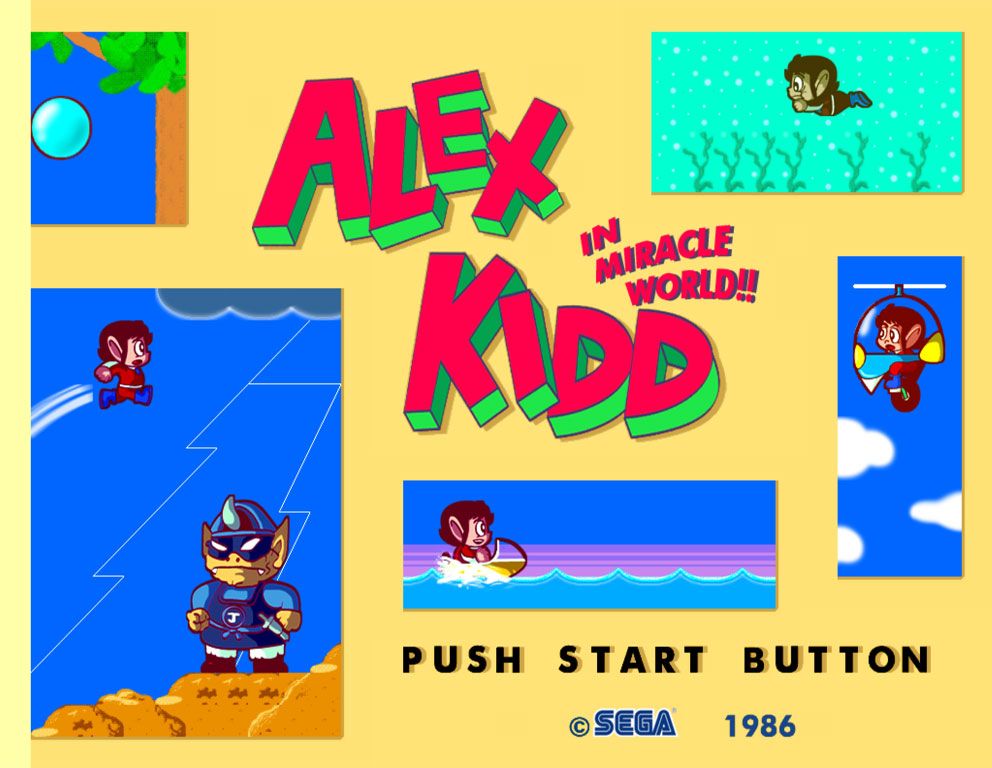
Original screen:
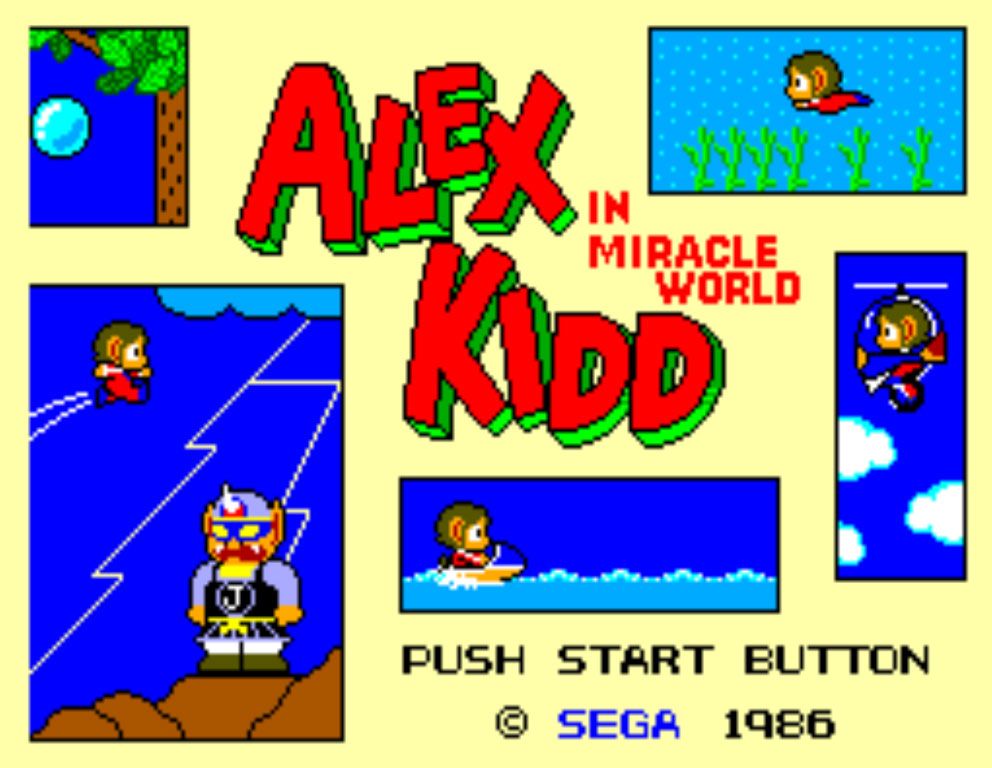
Very good work! Did you draw the sprites yourself?
I tried HiSMS, but I believe the interface could be better.
joabfarias wrote:
Very good work! Did you draw the sprites yourself?
I tried HiSMS, but I believe the interface could be better.
Thank you!

Yes, I'm drawing everything. The logo was heavily inspired from "Complete Album" cover (
http://www.jap-sai.com/Games/Alex_Kidd_ ... m_CD_A.jpg ) but I'm tracing / drawing / retouching every element on screen.
You should try this emulator again. It's very simple to use and it runs my modified game as fast as the original Alex Kidd.
There are a lot of features to be added or fixed (it's version 0.1 after all) but it's still an incredible software in my opinion.
Do you know how HiSMS works in palette changes? Do we need to make a HD version of the same sprite when it changes colors?
joabfarias wrote:
Do you know how HiSMS works in palette changes? Do we need to make a HD version of the same sprite when it changes colors?
Apparently yes.
I'm already facing this problem (Alex Kidd logo starts to blink after a few seconds -- and it suddenly goes back to the original 8-bit form when it happens).
It means that a version of MegaMan in a emulator that works like HiSMS would be a little bit boring to make...
Every single animation frame would have to be done a dozen times or more.
How dows HiSMS work when the sprite is partially hidden (like when Alex is behind bushes, for example)?
joabfarias wrote:
It means that a version of MegaMan in a emulator that works like HiSMS would be a little bit boring to make...
Every single animation frame would have to be done a dozen times or more.
Yep. But I still would do it if someone releases something like a "HiNES".

joabfarias wrote:
How dows HiSMS work when the sprite is partially hidden (like when Alex is behind bushes, for example)?
I'm not there yet but it's explained here:
http://hisms.orgfree.com/tutorial/index.html
joabfarias wrote:
It means that a version of MegaMan in a emulator that works like HiSMS would be a little bit boring to make...
Every single animation frame would have to be done a dozen times or more.
There are two ways to handle this:
- Have the emulator always use a constant palette that includes light, medium, and dark versions of all three colors in a particular sprite palette, as well as black, gray, and white.
- Use the palette changes as an opportunity to add unique detailing to Mega Man's sprite for each state.
Quote:
How dows HiSMS work when the sprite is partially hidden (like when Alex is behind bushes, for example)?
I imagine that most games just use the tiles' priority bits. (The SMS does it backward compared to the NES. On the SMS, priority is assigned to tiles, not sprites.) Games that perform occlusion in software using CHR RAM updates, like Solstice for NES, would have a bigger problem.
I still think the best possible approach is game-specific, as then the emulator can utilize the specifics of the game state in order to generate the appropriate graphics independently from the original tile-based approach. This would also allow the approach of using a completely different method of rendering, such as the 2.5D idea I mentioned. I'm not even sure how much more work it would be to do something like this as opposed to a generic "high-res tile" approach, although the latter would be easier for non-programmers to implement (but also more limited). For example, I'd love to see a SMB emulator that renders the graphics in the style of NSMB, but running the original game logic so all the known bugs would still be present (hat stomp, mushroom jump, wall anti-ejection, world 36). This would also present an interesting situation where existing TAS movies could be replayed on such an emulator to present a new and fresh look to something that is already very entertaining from a gameplay perspective.
I am trying to talk to Quietust (author of Nintendulator), at first he said that doubling the pixels directly in ROM and PPU (as we want to do) would not be feasible in Nintendulator because of the low level emulation of the PPU that Nintendulator uses.
Do any of you guys know what version of Visual Studio must I use to edit and run the source code of Nintendulator? I am using Visual Studio 2010 Ultimate, but I get errors when opening the project.
I was thinking in using a tecnique similar to the one used in HiSMS (sprite replacement), but instead of looking for specific colors, consider the sprite as it is (with four "indications" of color, 00, 01, 10 and 11). At the moment when the new sprite would be redraw, it would be done using the palette of the game. If it works, we will be able to avoid the palette changing issue.
joabfarias wrote:
Do any of you guys know what version of Visual Studio must I use to edit and run the source code of Nintendulator? I am using Visual Studio 2010 Ultimate, but I get errors when opening the project.
Works here with VS2010. You'll need the DirectX SDK for dxguid.lib.
I'm still praying for a "HiNES".
Meanwhile the first stage of my Alex Kidd HD prject is finally done:
http://www.youtube.com/watch?v=ScyB69dwRA0I *REALLY* would enjoy to recreate some NES classics to 720p.
All this talk reminds me of
Pacifi3D.
Code:
I still think the best possible approach is game-specific, as then the emulator can utilize the specifics of the game state in order to generate the appropriate graphics independently from the original tile-based approach. This would also allow the approach of using a completely different method of rendering, such as the 2.5D idea I mentioned. I'm not even sure how much more work it would be to do something like this as opposed to a generic "high-res tile" approach, although the latter would be easier for non-programmers to implement (but also more limited). For example, I'd love to see a SMB emulator that renders the graphics in the style of NSMB, but running the original game logic so all the known bugs would still be present (hat stomp, mushroom jump, wall anti-ejection, world 36). This would also present an interesting situation where existing TAS movies could be replayed on such an emulator to present a new and fresh look to something that is already very entertaining from a gameplay perspective.
I too have thought about this idea from time to time... but while it is an intriguing concept, both the effort involved and possible support it would gain make me question the success it might have. The main problem is the fact that you already mention: that the best possible approach is game specific. What we are really talking here is not really extending an emulator with some extra functions, but more about putting an emulator inside a highly flexible game engine having support for high-level scripting and all sorts of 3d content management. A creative artist retracing blocky graphics to bigger resolution sis a fairly simple task, but for this you would need a programmer with the knowledge, time and motivation to reverse engineer the NES *and* 3d graphics artists that can provide him/her with content for the sophisticated 3d engine.
I'm not saying it can't be done... but if anyone feels up to the task, I really think that the NES emulation part will be the very *least* of the troubles, so with all respect I kind of doubt this is the right forum to gather support for such a project. Better look around in general game programming forums for people who'd fancy doing truthful HD remakes of NES games.
Also, I'd say a multi-emulator system such as MESS would be a more likely candidate to shove into a modern 3d engine than any of the NES emulators discussed earlier, since that would give a bigger target audience and really, emulating the hardware perfectly is by definition not necessary.

I also once had this idea. Rice's plugin does something similiar for n64, it dumps textures to a folder -those can be edited and then get loaded when you run the game.
Raine emu also does something similar with a few arcade games.
If something like this could be applied to nes, maybe an emu with a tile dumper, then when the game loads it uses those tiles.
Maybe take it another step and 2x the tiles, and adjust the screen w/h. Having tiles 2x in size would really make it easier to make amazing looking edited sprites.
Possible? what do you guys think?
net44 wrote:
I also once had this idea. Rice's plugin does something similiar for n64, it dumps textures to a folder -those can be edited and then get loaded when you run the game.
Raine emu also does something similar with a few arcade games.
If something like this could be applied to nes, maybe an emu with a tile dumper, then when the game loads it uses those tiles.
Maybe take it another step and 2x the tiles, and adjust the screen w/h. Having tiles 2x in size would really make it easier to make amazing looking edited sprites.
Possible? what do you guys think?
Welcome Net44!
I think it would be perfect if all hi-res images could be stored directly into modified NES ROMs (instead of having them as separate bitmap files on a folder).
HiSMS emulator creates ROMs in a specific format (.hms) that put everything in a single file. It's amazing.
I keep hacking Master System games to high definition (like
http://youtu.be/XrqNfv3qiHA and
http://www.youtube.com/watch?v=FeegmIt03nM ) mostly because there's no (easy) way to do it to NES titles.