Just for fun, I decided to try what kind of an NES palette I would get by capturing from my el cheapo USB capture stick (Terratec Cinergy Hybrid T USB XS). I wrote a ROM to display all of the colors one after another, ran the ROM on my NTSC NES and PAL NES, captured a video with VirtualDub, and ran an AviSynth script to generate a palette picture.
Capture format was YUY2 (the stick doesn't support anything else), losslessly compressed with Lagarith (probably didn't help much). Settings (brightness/contrast/hue/saturation/sharpness) were at defaults.
ROM + source for ASM6 is attached (read the source header for usage instructions). The captured videos are pretty big (~50 MB) so I won't put them here.
Here are the palettes I got:
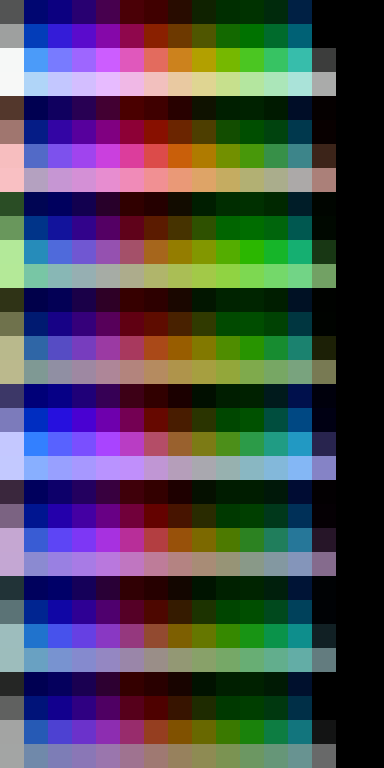
ntsc.png [ 4.06 KiB | Viewed 7537 times ]
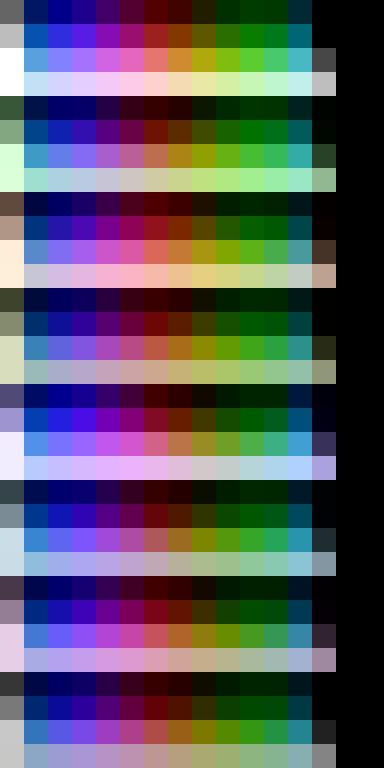
pal.png [ 4.08 KiB | Viewed 7537 times ]
AviSynth script: (If somebody else wants to use this, you will need to tweak a thing or two.)
Capture format was YUY2 (the stick doesn't support anything else), losslessly compressed with Lagarith (probably didn't help much). Settings (brightness/contrast/hue/saturation/sharpness) were at defaults.
ROM + source for ASM6 is attached (read the source header for usage instructions). The captured videos are pretty big (~50 MB) so I won't put them here.
Here are the palettes I got:
Attachment:
ntsc.png [ 4.06 KiB | Viewed 7537 times ]
Attachment:
pal.png [ 4.08 KiB | Viewed 7537 times ]
AviSynth script: (If somebody else wants to use this, you will need to tweak a thing or two.)
Code:
# c = AVISource( "ntsc-nes-full-palette.avi" )
c = AVISource( "pal-nes-full-palette.avi" )
# CONFIG: Use TFF or BFF depending on input video. If the colors aren't laid
# out properly, you picked the wrong one. "Properly" means gray in the upper
# left corner, then blue, and so on.
c = c.AssumeTFF()
# c = c.AssumeBFF()
# Remove bogus deinterlacing.
c = c.SeparateFields()
# Trim to only the relevant frames.
# CONFIG: Set the first (gray) frame.
# kFirstFrame = 47 # ntsc-nes-full-palette.avi
kFirstFrame = 48 # pal-nes-full-palette.avi
c = c.Trim( kFirstFrame, kFirstFrame+511 )
# Convert to RGB to have a common colorspace to work in independent of the
# input video format.
# \todo Not sure if there are downsides to doing this conversion?
c = c.ConvertToRGB()
# Crop to remove some crap from the borders.
kResultSize = 128
kCropHorz = (c.Width() - kResultSize)/2
kCropVert = (c.Height() - kResultSize)/2
c = c.Crop( kCropHorz, kCropVert, -kCropHorz, -kCropVert )
# Average the result by resizing down.
# \note Resizing to less than 4x4 produces an error.
# \todo Not really sure how good of a job this really does of averaging
# all the colors.
c = c.BicubicResize( 4, 4 )
# Crop to actually go down to 1x1.
c = c.Crop( 2, 2, -1, -1 )
# Convert to a single 16x32 frame.
c = c.WeaveColumns( 16 )
c = c.WeaveRows( 32 )
# Resize the palette elements up.
kPaletteElemSize = 24
c = c.PointResize( c.Width()*kPaletteElemSize, c.Height()*kPaletteElemSize )
c
c = AVISource( "pal-nes-full-palette.avi" )
# CONFIG: Use TFF or BFF depending on input video. If the colors aren't laid
# out properly, you picked the wrong one. "Properly" means gray in the upper
# left corner, then blue, and so on.
c = c.AssumeTFF()
# c = c.AssumeBFF()
# Remove bogus deinterlacing.
c = c.SeparateFields()
# Trim to only the relevant frames.
# CONFIG: Set the first (gray) frame.
# kFirstFrame = 47 # ntsc-nes-full-palette.avi
kFirstFrame = 48 # pal-nes-full-palette.avi
c = c.Trim( kFirstFrame, kFirstFrame+511 )
# Convert to RGB to have a common colorspace to work in independent of the
# input video format.
# \todo Not sure if there are downsides to doing this conversion?
c = c.ConvertToRGB()
# Crop to remove some crap from the borders.
kResultSize = 128
kCropHorz = (c.Width() - kResultSize)/2
kCropVert = (c.Height() - kResultSize)/2
c = c.Crop( kCropHorz, kCropVert, -kCropHorz, -kCropVert )
# Average the result by resizing down.
# \note Resizing to less than 4x4 produces an error.
# \todo Not really sure how good of a job this really does of averaging
# all the colors.
c = c.BicubicResize( 4, 4 )
# Crop to actually go down to 1x1.
c = c.Crop( 2, 2, -1, -1 )
# Convert to a single 16x32 frame.
c = c.WeaveColumns( 16 )
c = c.WeaveRows( 32 )
# Resize the palette elements up.
kPaletteElemSize = 24
c = c.PointResize( c.Width()*kPaletteElemSize, c.Height()*kPaletteElemSize )
c