Deleted. Nothing to see here. Google ReadNES3.
My code review comments:
Ordinarily, I'd allocate space for the header like this:
Code:
unsigned char headerData[16];
This would let me do one fread() to get the array.
I'd call memcmp() to compare the NES^Z signatures.
I'd use strncpy() to fill the HEADER instead of setting each character. On a PC, don't be afraid to make char arrays as long as you need.
You can declare a variable-sized array with malloc(); just make sure to destroy it with free() later.
Code:
unsigned char *bankCopyBuffer = malloc(datasize);
/* Omitted: do stuff with bankCopyBuffer */
free(bankCopyBuffer);
bankCopyBuffer = NULL;
Well I tried to add that but it kept erroring -.- For the sake of being lazy I guess I will just leave it like that. If anything, it saves space from including string.h, too.
Also I forgot to mention, it will only run by its self and only split a file named "Kaboom!.nes" unless you change it.....I may make it a click to run, then you enter the file in it that you want to make the ROM's for or make it run from a batch somehow. I really just made this for you guys to use if someone would need one since readNES I (tried to)use didn't work. I hope someone can find it useful! I got my game demo,Battle Kid Demo, Defender II and Mike Tyson: IPP to all split correctly so I guess everything is working as it should. I need to also add something to tell the use the program fails on fopen or fclose as others seem to think that it is #1 priority while I believe if it fails your gona know somehow

^_^
Kay well back to my learning in C land.....That was my first pile of crap ever so I think I am learning pretty darn quick.
I will not comment on the usage of the language's features because I know you are just getting started with C (I too usually can't get the most out of the language), but I will suggest you pay attention to a couple of bad programming practices before you make a habit out of them:
1. Indent your code: we usually don't do it in assembly because jumps and branches make logic blocks harder to distinguish, but there is no excuse in high level languages, which are "blocky" by nature. It might not make much difference for a simple and small program like this, but once you have loops within loops within loops (...) it becomes hell if it's not properly indented.
2. pick a naming convention: select conventions (preferably the ones that are already consolidated for the language) for naming your identifiers and stick to them. You have some variables with the first letter in caps and others that are all in caps. Why is that? Are they different in any way? Because the difference in the way they are written suggests that. Usually, all caps are used for constants. If you are not gonna follow the universal conventions (understandable, since you might not want to waste time researching that), at least create some you can obey.
As for the "Game.nes" thing, it's really easy to use parameters supplied by the user. Look into argc and argv and you'll see how easy it is to use a name supplied by the user, instead of hardcoding it to "Game.nes" (this will also allow users to drag and drop the ROM on your program, making it very simple to use). About the output files, you could either hardcode them to something like "character.chr" and "program.prg" or do some light string manipulation and use the same name of the input file and change only the extension to "chr" and "prg".
I was thinking like "Input .NES file to convert" prompt at the begining so you could have multiple NES files and just get the ones you need.....And yeah that will come next this is very useful though I don't wana keep it

ReadNES I used didn't ever work at all, it got annoying. I don't know of any other programs to extract those files so I just made a crappy one

Well, I don't know much about C myself, but this seems like a good alternative to dumb "hello world" programs to start out. I don't know how to acess hard drive in C or C++ I only know how to do this in java, so maybe your code will help me if I take the time to invest it (I'm busy with something else right now).
Like you say yourself, it's surprising such a "standard" language is such crippled. I've never been much a fan of C or C++ because every time you do a syntax error it's hell to fix it. Pointers and makefiles is also two major headaches that always kept me away of C/C++.
In Java, every time you do an error, eclipse automatically points it (you don't even need to save compile or whatever), and in 99% of cases it is really clear what error you made. You don't spend a weekend finding where you forget a ; or a } or something that would always happen in C or C++.
The reason C / C++ is yet so popular is probably speed/memory usage which is much better than all other high levels languages I think - but nothing will beat assembly here hehe.
I also know some Pascal/Delphi, but it is absolutely horrible you have to write chains and chains of "begin end" instead of {} which is stupid and annoying. It's used a little here because I think the author of the language is from my country, but that don't make the language great, that is for sure.
There is also a lot of other languages (Basic, Phyton, Pearl, C#, many others) I don't know anything about. Anything that is interpreted and not compiled will never be efficient tough.
65024U wrote:
I was thinking like "Input .NES file to convert" prompt at the begining
Don't do that... No good tool does that, only typical student programs ask users to type stuff at runtime, and that's really annoying! This prevents people from automating the tasks with batch files. Trust me, just use command line parameters. This way everyone is happy, the ones that want to type (and will do it in the command line) and the ones that want to automate (and will use batch files).
tokumaru wrote:
Trust me, just use command line parameters. This way everyone is happy, the ones that want to type (and will do it in the command line)
I agree for tools intended for use by programmers or by front-ends. But someone who uses Windows Explorer to open a program that takes command-line arguments will see a short usage message in a black window, and then the window disappears before anyone can read it. Most non-programmers I've met don't know how:
- to start Windows Command Prompt,
- to traverse the directory tree with 'cd' to get to the folder in question, as Windows doesn't come with an Open Command Prompt in This Folder command out of the box, or
- to enter a path with tab completion.
Do most non-programmers you've met know how to read a text file with instructions and drag-and-drop files? Then they'll be OK if the only argument is the source file. Most non-programmers wouldn't even have much use for tools like these anyway.
tokumaru wrote:
Most non-programmers wouldn't even have much use for tools like these anyway.
I guess my conclusion is biased by my experience in developing and supporting
GSM Player and
smsabuild, a command-line tool that I
tried to make easy for the GBA-flash-cart-owning public to use. But I'm not perfect. Someone using Windows ME
complained that he couldn't get the Windows 2000/XP version of GSM Player's batch file to work when the Windows 9x/ME version was sitting right next to it.
And about the latter, in
this post, Dwedit wrote:
Throw in directory traversal for a default named rom directroy, then you got a potentially useful utility, even usable by people who never touch command lines. Otherwise, specifying individual files at a command line is cumbersome to use.
65024U wrote:
String manipulation is a nightmare and declaring variables kinda weird at first.
String manipulation with pure C is not the way to go. When you need to write a small program that can parse complex text/code/strings, look into regexpes...
...and Perl.
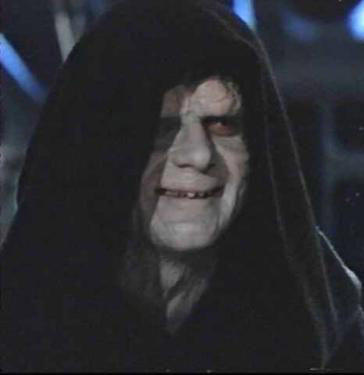
InvalidComponent wrote:
String manipulation with pure C is not the way to go.
Unless you're programming with Flex and Bison.
Quote:
When you need to write a small program that can parse complex text/code/strings, look into regexpes
Agreed.
Quote:
...and Perl.
You misspelled Python.
Just letting you know: If this becomes a scripting language holy war, I'll split it.
tepples wrote:
InvalidComponent wrote:
When you need to write a small program that can parse complex text/code/strings, look into regexpes
Agreed.
Quote:
...and Perl.
You misspelled Python.
Just letting you know: If this becomes a scripting language holy war, I'll split it.
Haha that is the funniest thing I've heard all day.
Okay well I will try to make it run from command line. With my command line programs I use batch files, those are really helpful. For NESASM3 I have the program, batch file, and assembly files all ine one, click the batch and it fires up and it's done super quick. Well lets go back and try to add this mysterious command line input....
Edit: Also breglad, I thought that too but now that I am getting into it, it's understandable but still sort of confusing, plus I gotta learn/find what I need in all these darn include .h files T.T I opened up ReadNES2 and I almost just closed it, it was insane 0_0
Perl vs. Python has been
splitted.
Okay guys I am learning alot more, I changed it so it basicly has to be run from command line with a file name included. It now tells you if it errors when opening files and yeah.....To run it set up a batch file
ReadNES2 *game namefilehere*.nes
pause
It will run and make the files, so anything else I should add? When I am done with it and satisfied, will you guys host it? It's only like a 4K program XD
HUGE update. New code, new feature (for this).....do what you like with it.
Yet again, you IRC guys are geniuses. Without you this'd have never been touched and I'd have probably stayed away from C for as long as possible but you helped so much.