So I've been trying and trying to implement a VWF for the dialogue in Furry RPG, but without success so far.
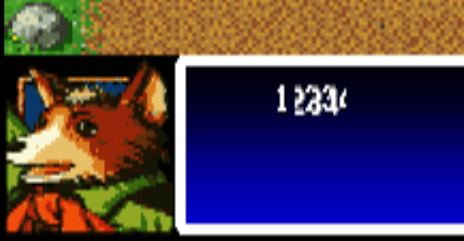
Note that I spent the last two weeks studying VWF source examples on RHDN (including this one) -- most of which are a pain to even read, or simply incomplete. Also, I stumbled across Stealth Translation's doc, and couldn't help but notice that it too is more than incomplete. Also, I looked at both tepples' Action menu and bisqwit's Chronotools 8×8 VWF engine, both of which only managed to confuse me even more.
Please note that the bit-shifting apparently works correctly -- the left half of the "2" appears shifted to the right just fine (albeit on the wrong tile), whereas the right half appears unnecessarily shifted, and shows up as garbage in an unwanted place.
Here's the code I came up with after studying all those other documents. Code executes during Vblank, variables starting with DP_ are direct page, vars starting with ARRAY_ are in the range of $0200-$1FFF (minus stack pointer) in WRAM:
Download:
http://manuloewe.de/snestuff/projects/furryrpg_build_00224.7z
Any substantial help is very much appreciated. Thanks!
Ramsis

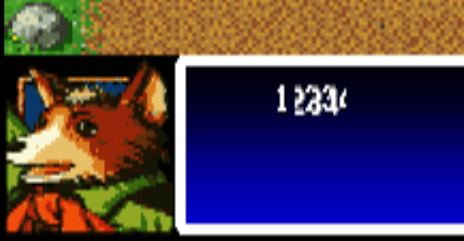
Note that I spent the last two weeks studying VWF source examples on RHDN (including this one) -- most of which are a pain to even read, or simply incomplete. Also, I stumbled across Stealth Translation's doc, and couldn't help but notice that it too is more than incomplete. Also, I looked at both tepples' Action menu and bisqwit's Chronotools 8×8 VWF engine, both of which only managed to confuse me even more.

Please note that the bit-shifting apparently works correctly -- the left half of the "2" appears shifted to the right just fine (albeit on the wrong tile), whereas the right half appears unnecessarily shifted, and shows up as garbage in an unwanted place.

Here's the code I came up with after studying all those other documents. Code executes during Vblank, variables starting with DP_ are direct page, vars starting with ARRAY_ are in the range of $0200-$1FFF (minus stack pointer) in WRAM:
Code:
ProcessVWFTiles:
A16
lda DP_TextASCIIChar ; ASCII char no. --> font tile no.
asl a ; value * 16 as 1 font tile = 16 bytes
asl a
asl a
asl a
tax
A8
lda DP_VWFBitsRemaining
and #$07
sta DP_VWFBitsRemaining
bne __UseOldVWFTile
A16
lda DP_VWFTileNew ; new tile no. --> VWF buffer index
bra +
__UseOldVWFTile:
A16
lda DP_VWFTileOld ; old tile no. --> VWF buffer index
+ asl a
asl a
asl a
asl a
tay
A8
lda #16 ; loop through 16 bytes per tile
sta DP_VWFLoop
- lda.l GFX_FontMode5, x
xba ; move to high byte
lda #$00
jsr __VWFShiftBits ; shift tile data if necessary
ora ARRAY_VWFTileBuffer1+1, y
sta ARRAY_VWFTileBuffer1+1, y
xba
ora ARRAY_VWFTileBuffer1, y
sta ARRAY_VWFTileBuffer1, y
iny
inx
dec DP_VWFLoop
bne -
ldx DP_TextASCIIChar ; ASCII char no. --> font width table index
lda DP_VWFBitsRemaining ; adjust pixels remaining for shifting
bne +
inc DP_VWFTileOld
inc DP_VWFTileNew
inc DP_VWFTileNew
lda #8 ; 8 = max. no. of bits
+ sec
sbc.l SRC_FWTDialogue, x
bpl +
eor #$FF ; handle overflow
inc a
sta DP_VWFBitsRemaining
inc DP_VWFTileOld
inc DP_VWFTileNew
inc DP_VWFTileNew
lda #8
sec
sbc DP_VWFBitsRemaining
+ sta DP_VWFBitsRemaining
rts
__VWFShiftBits:
phx
A16
pha
lda DP_VWFBitsRemaining
bne +
pla
bra __VWFShiftBitsDone
+ dec a
asl a
tax
pla
jmp (__VWFShiftAmount, x)
__VWFShiftAmount:
.DW _R1, _R2, _R3, _R4, _R5, _R6, _R7
_R1:
lsr a
_R2:
lsr a
_R3:
lsr a
_R4:
lsr a
_R5:
lsr a
_R6:
lsr a
_R7:
lsr a
__VWFShiftBitsDone:
A8
plx
rts
A16
lda DP_TextASCIIChar ; ASCII char no. --> font tile no.
asl a ; value * 16 as 1 font tile = 16 bytes
asl a
asl a
asl a
tax
A8
lda DP_VWFBitsRemaining
and #$07
sta DP_VWFBitsRemaining
bne __UseOldVWFTile
A16
lda DP_VWFTileNew ; new tile no. --> VWF buffer index
bra +
__UseOldVWFTile:
A16
lda DP_VWFTileOld ; old tile no. --> VWF buffer index
+ asl a
asl a
asl a
asl a
tay
A8
lda #16 ; loop through 16 bytes per tile
sta DP_VWFLoop
- lda.l GFX_FontMode5, x
xba ; move to high byte
lda #$00
jsr __VWFShiftBits ; shift tile data if necessary
ora ARRAY_VWFTileBuffer1+1, y
sta ARRAY_VWFTileBuffer1+1, y
xba
ora ARRAY_VWFTileBuffer1, y
sta ARRAY_VWFTileBuffer1, y
iny
inx
dec DP_VWFLoop
bne -
ldx DP_TextASCIIChar ; ASCII char no. --> font width table index
lda DP_VWFBitsRemaining ; adjust pixels remaining for shifting
bne +
inc DP_VWFTileOld
inc DP_VWFTileNew
inc DP_VWFTileNew
lda #8 ; 8 = max. no. of bits
+ sec
sbc.l SRC_FWTDialogue, x
bpl +
eor #$FF ; handle overflow
inc a
sta DP_VWFBitsRemaining
inc DP_VWFTileOld
inc DP_VWFTileNew
inc DP_VWFTileNew
lda #8
sec
sbc DP_VWFBitsRemaining
+ sta DP_VWFBitsRemaining
rts
__VWFShiftBits:
phx
A16
pha
lda DP_VWFBitsRemaining
bne +
pla
bra __VWFShiftBitsDone
+ dec a
asl a
tax
pla
jmp (__VWFShiftAmount, x)
__VWFShiftAmount:
.DW _R1, _R2, _R3, _R4, _R5, _R6, _R7
_R1:
lsr a
_R2:
lsr a
_R3:
lsr a
_R4:
lsr a
_R5:
lsr a
_R6:
lsr a
_R7:
lsr a
__VWFShiftBitsDone:
A8
plx
rts
Download:
http://manuloewe.de/snestuff/projects/furryrpg_build_00224.7z
Any substantial help is very much appreciated. Thanks!

Ramsis