Hello everybody. Thanks to bunnyboys nerdy nights over at NA, I've been doing a attempt at homebrewing.
The tutorials started with how to make a PONG game. I started from there and made a closed area where the ball keeps bouncing around.
The speed of my ball is adjustable (1-6). I can adjust this speed with the UP and Down buttons.
To display the speed I changed the background tile in the NMI. This semi-works. Before I change the speed (up or down), it displays 1.
After I change the speed, it shoves the "1" one place to the right and displays the actual speed (1 to 6).
This happens only one time. The number 1 just shoves over to the right and the new number is adjustable (changing the speed - as intended)
Anyone have any idea what's wrong ? Here is my code for displaying the speed: http://nintendoage.pastebin.com/f61fb15b5
The "Drawscore:" gets called upon in the NMI.
--------------
Also, would it be better if I removed the highlighted pieces of codes (in the pastebin) and add a Jump after a compare (if the compare = 1) ?
In the below example, first it would load A with the ball speed. If it's not equal to six, it will jump to the next value (NotSix). A is still loaded with the ball speed, so there is no need to load it again ?
If the value is equal to six, the Jump is then used to skip unnecessary code and to prevent that the tile number stored in A get's compared with other speeds (5-1)
So you would get:
Drawscore:
LDA #$23 ;load the first part of the location for the score
STA $2006 ; store into input port of PPU
LDA #$53 ;load the second part of the location for the score
STA $2006 ; store again
NotFive:
.....
.....
DrawScoreDone:
The tutorials started with how to make a PONG game. I started from there and made a closed area where the ball keeps bouncing around.
The speed of my ball is adjustable (1-6). I can adjust this speed with the UP and Down buttons.
To display the speed I changed the background tile in the NMI. This semi-works. Before I change the speed (up or down), it displays 1.
After I change the speed, it shoves the "1" one place to the right and displays the actual speed (1 to 6).
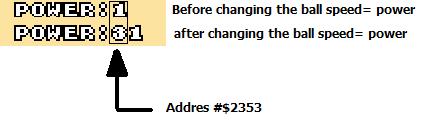
This happens only one time. The number 1 just shoves over to the right and the new number is adjustable (changing the speed - as intended)
Anyone have any idea what's wrong ? Here is my code for displaying the speed: http://nintendoage.pastebin.com/f61fb15b5
The "Drawscore:" gets called upon in the NMI.
--------------
Also, would it be better if I removed the highlighted pieces of codes (in the pastebin) and add a Jump after a compare (if the compare = 1) ?
In the below example, first it would load A with the ball speed. If it's not equal to six, it will jump to the next value (NotSix). A is still loaded with the ball speed, so there is no need to load it again ?
If the value is equal to six, the Jump is then used to skip unnecessary code and to prevent that the tile number stored in A get's compared with other speeds (5-1)
So you would get:
Drawscore:
LDA #$23 ;load the first part of the location for the score
STA $2006 ; store into input port of PPU
LDA #$53 ;load the second part of the location for the score
STA $2006 ; store again
- LDA ballspeedx
CMP #$06
BNE NotSix
LDA #$42
STA $2007
JMP DrawScoreDone ;if the speed of the ball is six => no need to do 5-1, jump to bottom
- LDA ballspeedx ; this rule gets deleted, because if it's not 6, no other value was stored in A
CMP #$05
BNE NotFive
LDA #$51
STA $2007
JMP DrawScoreDone
NotFive:
.....
.....
DrawScoreDone: