Hello,
I've been lurking here for a bit, learning 6502 and poking around at some disassemblies, working up to taking on a from-scratch or mod project at some point. In the process of doing so, I've been writing my own disassembler and tile editor to help pick apart existing ROMs. Although it might just be a throwaway tool, it might be useful to folks with some feedback and further development.
As far as the disassembler goes, rather than simply dump the entire contents of the ROM into what may or may not be valid 6502 code, it traces the program's execution and tries to identify subroutine and data table symbols to create some meaningful output. Getting it to handle jumptables was interesting, but there are still many more spots in code that mislead the program, particularly with bank switching. What I'd like to do is have it do a "best effort" and then allow the user to interactively continue the disassembly when it gets stuck, then automatically continue the disassembly until it stops again. Symbols will be able to be named something more meaningful than the default "SR_0F_C000" and such. With a completed disassembly, it'd be able to accept code/data changes and re-compile with its symbol table.
As for the tile editor side of the program, right now I've just got it reading from the ROM and displaying either PRG or CHR data on the left-hand column and displaying various patterns of tiles on the right-hand side with whatever palette is selected. It won't be long before it can edit and save changes to the tile data. Here's a preview of what it currently looks like...
So my question for all of you is, would a tool like I've described be useful, and what kind of features would you like to see? Thanks!
I've been lurking here for a bit, learning 6502 and poking around at some disassemblies, working up to taking on a from-scratch or mod project at some point. In the process of doing so, I've been writing my own disassembler and tile editor to help pick apart existing ROMs. Although it might just be a throwaway tool, it might be useful to folks with some feedback and further development.
As far as the disassembler goes, rather than simply dump the entire contents of the ROM into what may or may not be valid 6502 code, it traces the program's execution and tries to identify subroutine and data table symbols to create some meaningful output. Getting it to handle jumptables was interesting, but there are still many more spots in code that mislead the program, particularly with bank switching. What I'd like to do is have it do a "best effort" and then allow the user to interactively continue the disassembly when it gets stuck, then automatically continue the disassembly until it stops again. Symbols will be able to be named something more meaningful than the default "SR_0F_C000" and such. With a completed disassembly, it'd be able to accept code/data changes and re-compile with its symbol table.
As for the tile editor side of the program, right now I've just got it reading from the ROM and displaying either PRG or CHR data on the left-hand column and displaying various patterns of tiles on the right-hand side with whatever palette is selected. It won't be long before it can edit and save changes to the tile data. Here's a preview of what it currently looks like...
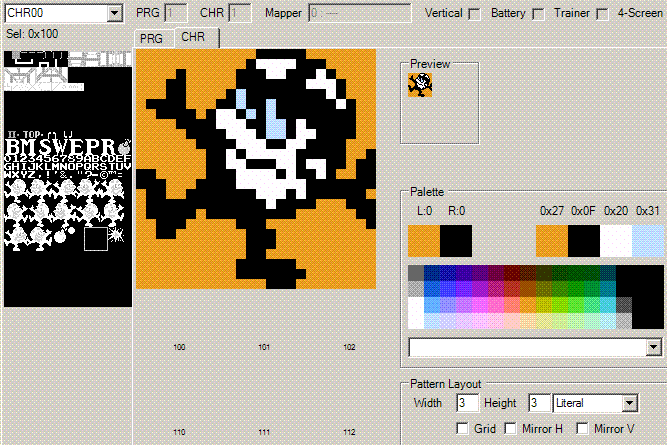
So my question for all of you is, would a tool like I've described be useful, and what kind of features would you like to see? Thanks!