RECCA is probably the best example of all that I'm going to ask here. Normally most of this stuff is really easy, but taking how limited the NES, is you don't have access to trigonometric functions which makes things a lot more complicated.
1. Aimed shots. I know a lot of games do this but I never understood how they do this. As a side thing, I'd also like to know how to add angular offset (as shown by the enemies in the picture below by shooting a 3-way shots).
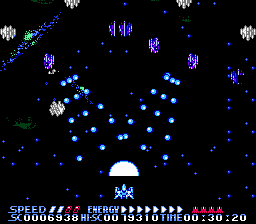
Summer Carnival '92 - Recca (Japan)-18.png [ 4.21 KiB | Viewed 2309 times ]
2. Homing shots. Somewhat common, but still a mystery in to me.
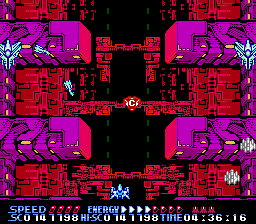
Summer Carnival '92 - Recca (Japan)-20.png [ 7.42 KiB | Viewed 2309 times ]
3. Angular velocity. RECCA also has an enemy that has other objects spinning around it, which I know for a fact that creating such movement requires changing the angle x amount of degrees every frame to make it seem like its turning.
*no pucture for this one :c
4. Bonus! I don't even know how to explain this. I'm talking about that 'hand' in the top left corner. It starts retracted(?) and starts to extend out and reach out towards the player. I can't even figure out how to program something like that with the mathematical capabilities modern languages have...
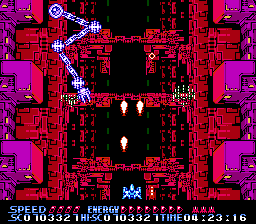
Summer Carnival '92 - Recca (Japan)-19.png [ 7.85 KiB | Viewed 2309 times ]
1. Aimed shots. I know a lot of games do this but I never understood how they do this. As a side thing, I'd also like to know how to add angular offset (as shown by the enemies in the picture below by shooting a 3-way shots).
Attachment:
Summer Carnival '92 - Recca (Japan)-18.png [ 4.21 KiB | Viewed 2309 times ]
2. Homing shots. Somewhat common, but still a mystery in to me.
Attachment:
Summer Carnival '92 - Recca (Japan)-20.png [ 7.42 KiB | Viewed 2309 times ]
3. Angular velocity. RECCA also has an enemy that has other objects spinning around it, which I know for a fact that creating such movement requires changing the angle x amount of degrees every frame to make it seem like its turning.
*no pucture for this one :c
4. Bonus! I don't even know how to explain this. I'm talking about that 'hand' in the top left corner. It starts retracted(?) and starts to extend out and reach out towards the player. I can't even figure out how to program something like that with the mathematical capabilities modern languages have...
Attachment:
Summer Carnival '92 - Recca (Japan)-19.png [ 7.85 KiB | Viewed 2309 times ]